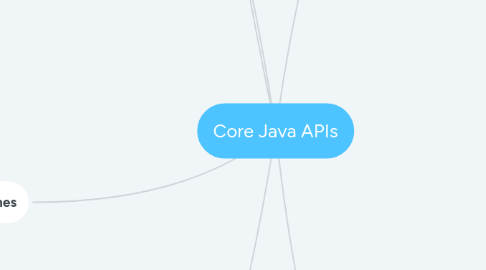
1. StringBuffer
1.1. thread safe
2. StringBuilder
2.1. Mutable
2.2. Methods
2.2.1. append()
2.2.2. charAt()
2.2.3. delete()
2.2.4. deleteCharAt()
2.2.5. indexOf()
2.2.6. insert()
2.2.7. length()
2.2.8. reverse()
2.2.9. substring()
2.2.10. toString()
2.2.11. equals()
2.2.11.1. check pointing to the same object and looking at the values inside
2.3. ==
2.3.1. check pointing to the same StringBuilder object
3. Array
3.1. Fixed-size area of memory
3.2. Methods
3.2.1. sort()
3.2.2. binarySearch()
3.2.2.1. sorted array
3.2.2.2. return the index of match
3.2.2.3. unsorted arry
3.2.2.3.1. unpredictable output
3.3. primitive
3.4. pointers to objects
3.5. Multidimensional
3.5.1. int[][] a;
3.5.2. int a[][];
3.5.3. int[] a[];
3.5.4. int[] a[][];
4. Date and Times
4.1. immutable
4.1.1. private constructor
4.1.1.1. LocalTime
4.1.1.1.1. Methods
4.1.1.1.2. LocalDate.now()
4.1.1.1.3. LocalDate.of()
4.1.1.2. LocalDateTime
4.1.1.3. LocalDate
4.2. Period
4.2.1. days
4.2.2. month
4.2.3. year
4.3. DateTimeFormatter
4.3.1. output format of date and time
4.3.2. DateTimeFormatter f = DateTimeFormatter.ofPattern("hh:mm");
4.3.3. M, m, h, y
4.3.3.1. M = Month
4.3.3.2. m = Minute
5. String
5.1. String pool
5.1.1. Literals
5.2. Methods
5.2.1. charAt()
5.2.2. concat()
5.2.3. endsWith()
5.2.4. equals()
5.2.4.1. check sequence of characters
5.2.5. equalsIgnoreCase()
5.2.6. indexOf()
5.2.7. length()
5.2.8. replace()
5.2.9. startsWith()
5.2.10. substring()
5.2.11. toLowerCase()
5.2.12. toUpperCase()
5.2.13. trim()
5.3. Immutable
5.4. ==
5.4.1. check point to the same object in the pool
5.5. public static void main
5.5.1. (String[] args)
5.5.2. (String args)
5.5.3. (String... args)
5.5.3.1. varargs
6. Collections
6.1. List
6.1.1. ArrayList
6.1.1.1. Dinamic size
6.1.1.2. List<Integer> h = new ArrayList<>();
6.1.1.3. Methods
6.1.1.3.1. add()
6.1.1.3.2. clear()
6.1.1.3.3. contains()
6.1.1.3.4. equals()
6.1.1.3.5. isEmpty()
6.1.1.3.6. remove()
6.1.1.3.7. set()
6.1.1.3.8. size()
6.1.1.4. not allowed to contain primitives
6.1.2. Vector
6.1.3. LinkedList