Software Design
by Chris Larson
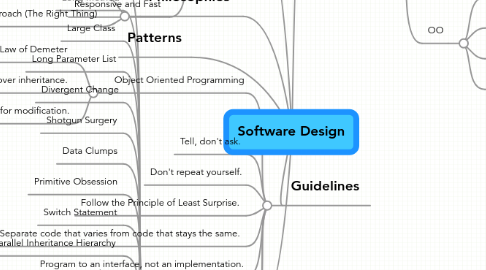
1. Goals
1.1. Scalable
1.2. User Friendly
1.2.1. Intuitive Interface
1.2.2. Well Documented
1.3. Maintainable
1.4. Feature Complete
1.5. Reliable
1.6. Responsive and Fast
2. Guidelines
2.1. Object Oriented Programming
2.1.1. Law of Demeter
2.1.2. Favor composition over inheritance.
2.1.3. Open-Closed Principle (OCP) - Classes should be open for extension, but closed for modification.
2.2. Tell, don't ask.
2.3. Don't repeat yourself.
2.4. Follow the Principle of Least Surprise.
2.5. Separate code that varies from code that stays the same.
2.6. Program to an interface, not an implementation.
2.7. You're Not Going To Need It (YAGNI) - Favors Simplicity over Completeness.
2.8. Design to avoid Rigidity, Fragility, and Immobility.
3. Philosophies
3.1. Worse is Better
3.2. MIT approach (The Right Thing)
4. Code Smells
4.1. Duplicated Code
4.2. Long Method
4.3. Large Class
4.4. Long Parameter List
4.5. Divergent Change
4.6. Shotgun Surgery
4.7. Data Clumps
4.8. Primitive Obsession
4.9. Switch Statement
4.10. Parallel Inheritance Hierarchy
4.11. Lazy Class
4.12. Eliminate unuseful classes
4.13. Speculative Generality
4.14. Temporary Field
4.15. Message Chains
4.16. Middle Man
4.17. Inappropriate Intimacy
4.18. Alternative Classes with different interfaces
4.19. Incomplete Library Class
4.20. Data Class
4.21. Refused Bequest
4.22. Comments
5. Patterns
6. Principles
6.1. Simplicity
6.2. Consistency
6.3. Completeness
6.4. Correctness
6.5. Efficiency
6.6. Clarity
6.7. Portability
6.8. Code Safety
6.9. Security
6.10. Intuitive
6.11. OO
6.11.1. Low Coupling
6.11.2. High Cohesion
6.11.3. Polymorphism
6.11.4. Encapsulation